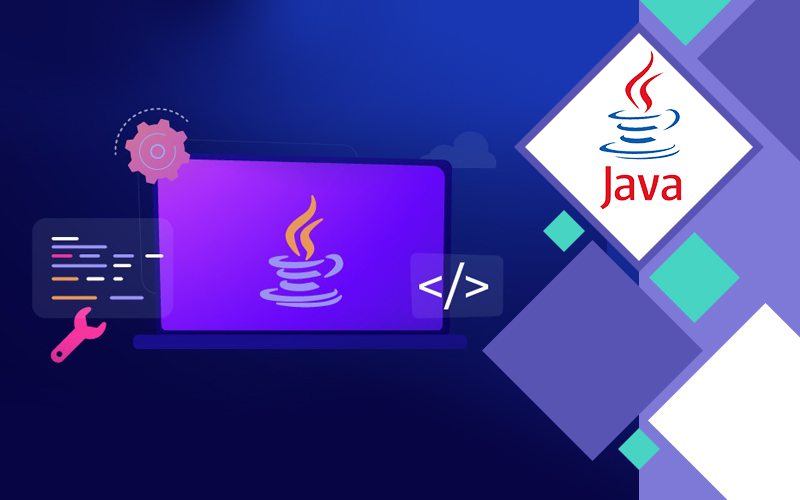
شرح دوره آموزش Java SE
دوره آموزش Java SE (Standard Edition) یک دوره جامع برای یادگیری زبان برنامهنویسی جاوا در سطح پایه و متوسط است. جاوا یکی از پرکاربردترین و قدرتمندترین زبانهای برنامهنویسی در جهان است که به دلیل پایداری، قابلیت اطمینان و انعطافپذیری، در توسعه برنامههای وب، موبایل و سیستمهای دسکتاپ استفاده میشود. نسخه استاندارد جاوا (Java SE) شامل کتابخانهها و ابزارهای اصلی است که هر برنامهنویس برای شروع یادگیری و توسعه برنامههای جاوا به آنها نیاز دارد.
مخاطبین:
- متخصصان توسعه نرم افزار
- مدیران و مشاوران پروژه های نرم افزاری
- متخصصین وب
نحوه برگزاری:
بصورت آنلاین و حضوری
مدت دوره :
60 ساعت
- Basics of Java
What is Java?
History and Features of Java
Hello Java Program
JDK, JRE, and JVM
JVM Memory Management
Internal details of JVM
Operators, Keywords, and Control Statements like if-else, switch, for loop, while loop, etc.
- Class, Object, Packages in Java
Naming convention of java
Classes, Objects, and Features.
Object declaration and initialization
Life cycle of an object
Declare package and Package naming conventions
Types of packages such as user-defined packages, built-in packages
Importing packages in Java
- Data types in Java
Primitive data types
Non-primitive data types
Memory allocation of primitive and non-primitive data types, etc.
Variables, Constants, and Literals
Types of variables such as local variables, instance variables, and static variables
Scope and memory allocation of variables.
- Method and Constructor in Java
Methods in Java
Use of method in Java
Method declaration, method signature
Types of methods in Java: predefined method, user-defined methods: instance method, static method
Java main method
Return type in Java
Block in Java
What is Constructor in Java and types of it?
Constructor chaining in java
- Modifiers in Java
Access modifier and Non-access modifier in Java
Types of access modifiers
Types of Non-access modifiers
- Static and Final Keywords
What is Static keyword?
Static methods, Static block, Static Nested Class in Java
Final keyword, Final variable, final method, Final class
- OOPs concepts
Class, Object, Encapsulation, Inheritance, Polymorphism, Abstraction
Types of inheritance
Abstract class vs interface
- Types of classes in java
What is inner class?
Nested, Inner, Local and Anonymous Classes
- Interfaces
Defining an Interface
Implementing Interfaces
Accessing Implementations Through Interface References
Partial Implementations
Nested Interfaces
Applying Interfaces
Variables in Interfaces
Interfaces Can Be Extended
Default Interface Methods
Static Interface Methods
Private Interface Methods
Private static Interface Methods
Multiple Inheritance Issues
Use static Methods in an Interface
Final Thoughts on Packages and Interfaces
- Functional programming, Lambda Expressions, Method Reference and Stream
Using Variables in Lambdas
Working with Built-In Functional Interfaces
Implementing Supplier
Implementing Consumer and BiConsumer
Implementing Predicate and BiPredicate
Implementing Function and BiFunction
Implementing UnaryOperator and BinaryOperator
Checking Functional Interfaces
Returning an Optional
Using Streams
Creating Stream Sources
Using Common Terminal Operations
Using Common Intermediate
Putting Together the Pipeline
Lambda Expression Fundamentals
Functional Interfaces
Some Lambda Expression Examples
Block Lambda Expressions
Generic Functional Interfaces
Passing Lambda Expressions as Arguments
Lambda Expressions and Variable Capture
Method References
Method References to static Methods
Method References to Instance Method
Method References with Generics
Constructor References
Predefined Functional Interfaces
- Java Annotations
Annotations (Metadata)
Annotation Basics
Specifying a Retention Policy
Obtaining Annotations at Run Time by Use of Reflection
Obtaining All Annotations
The Annotated Element Interface
Using Default Values in annotation
Marker Annotations
Single-Member Annotations
The Built-In Annotations
Introspection and Java Reflection API
- Garbage Collection
- Collections Framework
What is Collections Framework?
List, Set, Queue, Deque, Map, Iterator, and Enumeration.
ArrayList, LinkedList, HashSet, LinkedHashSet, TreeSet, ArrayDeque, PriorityDeque, EnumSet, SortedSet, AbstractList, HashMap, LinkedHashMap, TreeMap
Comparator
Comparable
- Serialization
Serialization, Deserialization, and Java transient keyword
- Exception Handling in Java
Exception Handling in Java
Try-catch block
Multiple Catch Block
Nested try block
Finally block
Throw Keyword
Throws Keyword
Throw vs Throws, Final vs Finally vs Finalize
Exception Handling with Method Overriding Java Custom Exceptions
- Reflection in Java
What are reflection concepts in Java?
Reflection API
NewInstance() & Determining the class object
Accessing private method from outside the class
- Java Thread
The Main Thread
Creating a Thread
Implementing Runnable
Extending Thread
Java Thread Model
Understanding Thread Concurrency
Synchronization and Locking
Thread Cache and volatile
Executor Service, Future and Thread Pools
Introducing the Single-Thread Executor
Shutting Down a Thread Executor
Submitting Tasks
Waiting for Results
Using isAlive( ) and join( )
Thread Priorities
Synchronization
Using Synchronized Methods
Timer, Scheduling and Re-Try
Shutdown Hooks and JVM Shutdown Sequence
- Input Output Stream
File
Temporary File
I/O Basics
Streams
InputStream
FileInputStream
FileOutputStream
BufferedInputStream
BufferedOutputStream
Byte Streams and Character Streams
The Predefined Streams
Reading Console Input
Reading Characters
Reading Strings
Writing Console Output
The PrintWriter Class
File Handling and Performance Issues
Regular Expressions
Writing Software Agents
Object Serialization and Versioning
XML Processing
XML Bindings and Marshaling using JAXB
JSON Processing Libs
Properties class and JVM Properties
Logging and Log Levels
- JDBC
JDBC Drivers
Steps to connect to Database
Connectivity with Oracle
Connectivity with MySQL
Connectivity with Access without DSN
DriverManager
Types of JDBC statements: Statement, Prepared statement, Callable statement
Database Metadata, Resultset Metadata
ResultSet, types of ResultSet,
Storing image, Retrieving image
Storing file, Retrieving file, Stored procedures, and functions
Transaction Management
Batch Processing
JDBC New Features, Mini Project, and interview questions.
- Agile
In this chapter, you will familiar with
Agile model
Advantages, and Disadvantages of Agile model
Agile versus Waterfall method
Important terminology: Scrum, Scrum Master, Flow of Agile Implementation, Sprint, and
Burn down Charts.
- Design Pattern
Singleton Object
Singleton design pattern with Serialization
Factory Pattern
Abstract Factory.
- Database or Overview of Java Persistence API (JPA)
درخواست مشاوره
برای کسب اطلاعات بیشتر درباره این دوره درخواست مشاوره خود را ارسال کنید و یا با ما در تماس باشید.
درخواست مشاورهدوره های مرتبط
دوره Java Spring Framework
دوره آموزشی Java Spring Framework مدت دوره : 70 ساعت شرح دوره : این دوره از جمله دوره های فوق…
نظرات
6,100,000 هزار تومان