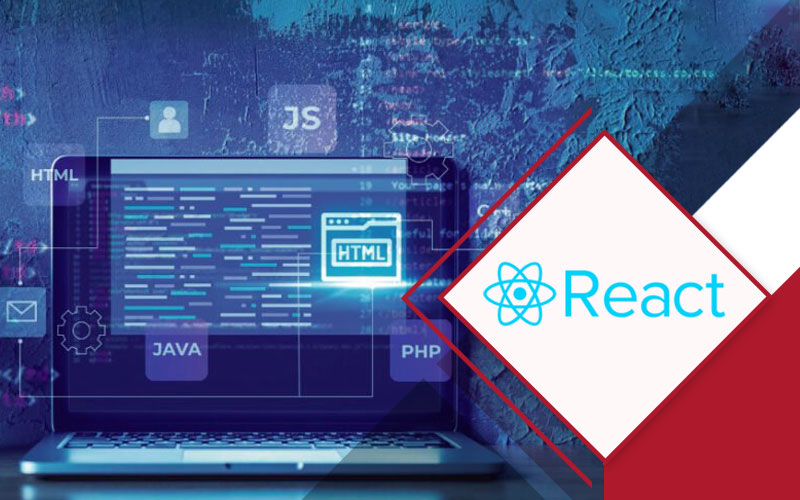
شرح دوره آموزش React
دوره آموزش React یک راهنمای جامع و عملی برای یادگیری یکی از محبوبترین کتابخانههای جاوااسکریپت در حوزه توسعه وب است. React، که توسط فیسبوک توسعه یافته است، به توسعهدهندگان اجازه میدهد تا رابطهای کاربری سریع، پویا و کاربرپسند بسازند. این دوره شما را با اصول اولیه React، از جمله ساختار کامپوننتها، مدیریت وضعیت (state)، و استفاده از Props آشنا میکند. همچنین، با یادگیری مفاهیم پیشرفتهتر مانند مدیریت رویدادها، چرخه حیات کامپوننتها، و کار با کتابخانههای مکمل مانند React Router و Redux، مهارت لازم برای ساخت برنامههای کاربردی تحت وب قدرتمند و مقیاسپذیر را به دست خواهید آورد.
این دوره برای توسعهدهندگان مبتدی و متوسط طراحی شده است و علاوه بر مباحث تئوری، شامل پروژههای عملی است تا بتوانید در حین یادگیری مهارتهای خود را به کار ببرید و با چالشهای دنیای واقعی مواجه شوید. در پایان این دوره، قادر خواهید بود وباپلیکیشنهای مدرن و تعاملی با استفاده از React ایجاد کنید و به دنیای توسعه وب حرفهای وارد شوید.
مخاطبان این دوره:
شامل توسعهدهندگان وبی با سطوح مختلف تجربه میشود، از مبتدیتا حرفهای. اگر شما در توسعه وب آشنایی دارید و میخواهید به صورت عمیقتر با React آشنا شوید یا مهارتهای خود را در این زمینه تقویت کنید، این دوره میتواند مناسب برای شما باشد. تدریس مفاهیم پایهای تا متوسط و پیشرفتهٔ React، مدیریت وضعیت، مدیریت روتینگ، و ارتباط با سرویسهها
Introduction to React (Duration: 2 hours)
- An overview of React and its features, including its component-based architecture and virtual DOM.
- Setting up the development environment with tools like Node.js, npm or Yarn, and a code editor.
- Creating a basic React project using Create React App or setting up a custom project structure.
- Understanding the role of Babel and Webpack in transpiling and bundling React applications.
- Exploring the React developer tools for browser debugging and performance analysis.
- Comparing React to other popular frameworks like Angular and Vue.js, highlighting its strengths and use cases.
React Fundamentals (Duration: 10 hours)
- Understanding the concept of components in React and their lifecycle methods.
- Exploring JSX syntax and how it allows mixing HTML-like code with JavaScript.
- Working with the virtual DOM and understanding its benefits for efficient rendering.
- Managing state in React components using the useState hook.
- Passing data between components using props.
- Handling user interactions and events with event handlers in React.
- Understanding the importance of keys in dynamic lists of components.
- Using conditional rendering to show or hide elements based on specific conditions.
- Exploring the role of React Fragments in rendering multiple components without an extra wrapper.
- Implementing controlled and uncontrolled forms in React.
Part 3: Building UI Components (Duration: 12 hours)
- Creating functional components and understanding their advantages.
- Styling React components using CSS-in-JS libraries like styled-components or Emotion.
- Implementing responsive design techniques using media queries and CSS frameworks like Bootstrap or Material-UI.
- Exploring popular UI component libraries for React, such as Material-UI or Ant Design.
- Creating reusable and composable components to improve code maintainability.
- Using React context for sharing data across components.
- Implementing compound components to encapsulate related functionality.
- Working with higher-order components (HOCs) and their role in component composition.
- Exploring React hooks beyond useState, such as useEffect for handling side effects.
Managing Data and State (Duration: 10 hours)
- Understanding state management options in React, including local component state and global state management libraries.
- Implementing more advanced state management with Redux or MobX.
- Making HTTP requests and handling asynchronous operations with Axios or Fetch.
- Working with RESTful APIs and handling data fetching and manipulation.
- Implementing forms with form validation using libraries like Formik or React Hook Form.
- Handling form submission and processing data on the server-side.
Routing and Navigation (Duration: 6 hours)
- Implementing client-side routing in React using React Router.
- Defining routes, route parameters, and nested routes.
- Implementing route guards to control access to certain routes.
- Navigating programmatically using history and link components.
- Implementing dynamic routing based on data or user input.
- Enhancing navigation with redirects and custom transitions between routes.
Optimizing Performance (Duration: 8 hours)
- Identifying performance bottlenecks in React applications.
- Using React.memo and useMemo for memoization to optimize rendering.
- Employing useCallback to optimize function references.
- Splitting code and lazy loading components using React.lazy and code splitting techniques.
- Using React Suspense to handle loading states and implement lazy loading.
- Implementing virtualized lists for efficient rendering of large datasets.
- Analyzing performance using the React Profiler tool.
Part 7: Testing and Debugging (Duration: 6 hours)
- Writing unit tests for React components using Jest and React Testing Library.
- Understanding testing principles such as test-driven development (TDD) and behavior-driven development (BDD).
- Mocking dependencies and simulating user interactions in tests.
- Debugging React applications using browser DevTools, inspecting component state, and debugging events.
- Analyzing performance using browser performance tools and React Profiler.
Advanced Topics (Duration: 16 hours)
- Server-side rendering (SSR) with React using frameworks like Next.js.
- Building progressive web applications (PWAs) with React and implementing offline capabilities.
- Integrating React with GraphQL using libraries like Apollo Client.
- Deploying React applications to cloud platforms like AWS, Azure, or Netlify.
- Exploring advanced React patterns like render props, context API, and custom hooks.
- Implementing internationalization and localization in React applications.
- Handling authentication and authorization in React applications.
درخواست مشاوره
برای کسب اطلاعات بیشتر درباره این دوره درخواست مشاوره خود را ارسال کنید و یا با ما در تماس باشید.
درخواست مشاورهدوره های مرتبط
دوره آموزش برنامه نویسی فرانت اند Front end
در دنیای دیجیتال امروز، طراحی و توسعه وب به یکی از مهارتهای کلیدی تبدیل شده است. دوره آموزش برنامهنویسی فرانتاند با هدف آشنایی شما با اصول و فنون ایجاد وبسایتهای جذاب و کاربرپسند طراحی شده است. در این دوره، شما با زبانهای اصلی برنامهنویسی مانند HTML، CSS و JavaScript آشنا خواهید شد و توانایی ساخت رابطهای کاربری زیبا و تعاملی را خواهید یافت.
ما به شما ابزارهای لازم برای توسعه وبسایتهای مدرن را آموزش خواهیم داد و نکات مهمی درباره بهینهسازی تجربه کاربری و طراحی ریسپانسیو را در اختیارتان قرار میدهیم. در پایان این دوره، شما با اعتماد به نفس میتوانید پروژههای فرانتاند خود را شروع کنید و به دنیای توسعه وب وارد شوید. بیایید با هم سفر یادگیری را آغاز کنیم!
دوره آموزش ASP.NET MVC
دوره آموزش ASP.NET MVC به یادگیری توسعه وباپلیکیشنها با استفاده از فریمورک ASP.NET MVC میپردازد. این دوره به شرکتکنندگان کمک میکند تا مهارتهای لازم برای طراحی و پیادهسازی وبسایتها و وباپلیکیشنهای دینامیک را یاد بگیرند.
آموزش مجموعه mern
مقدمه مجموعه mern اشاره به اول کلمه چهار دوره و تخصص (mongodb, express.js, react.js, node.js) mongodb برای مدیریت و کار…
دوره آموزش IOS Programming Advance
شرح دوره IOS Programming Advance
نظرات
4,500,000 هزار تومان